Intel 8086 Assembly: Architecture & Registry Explained
An introductory article to Intel 8086, the first x86 processor. We'll discuss the architecture, registries, flags, adressing and much more.
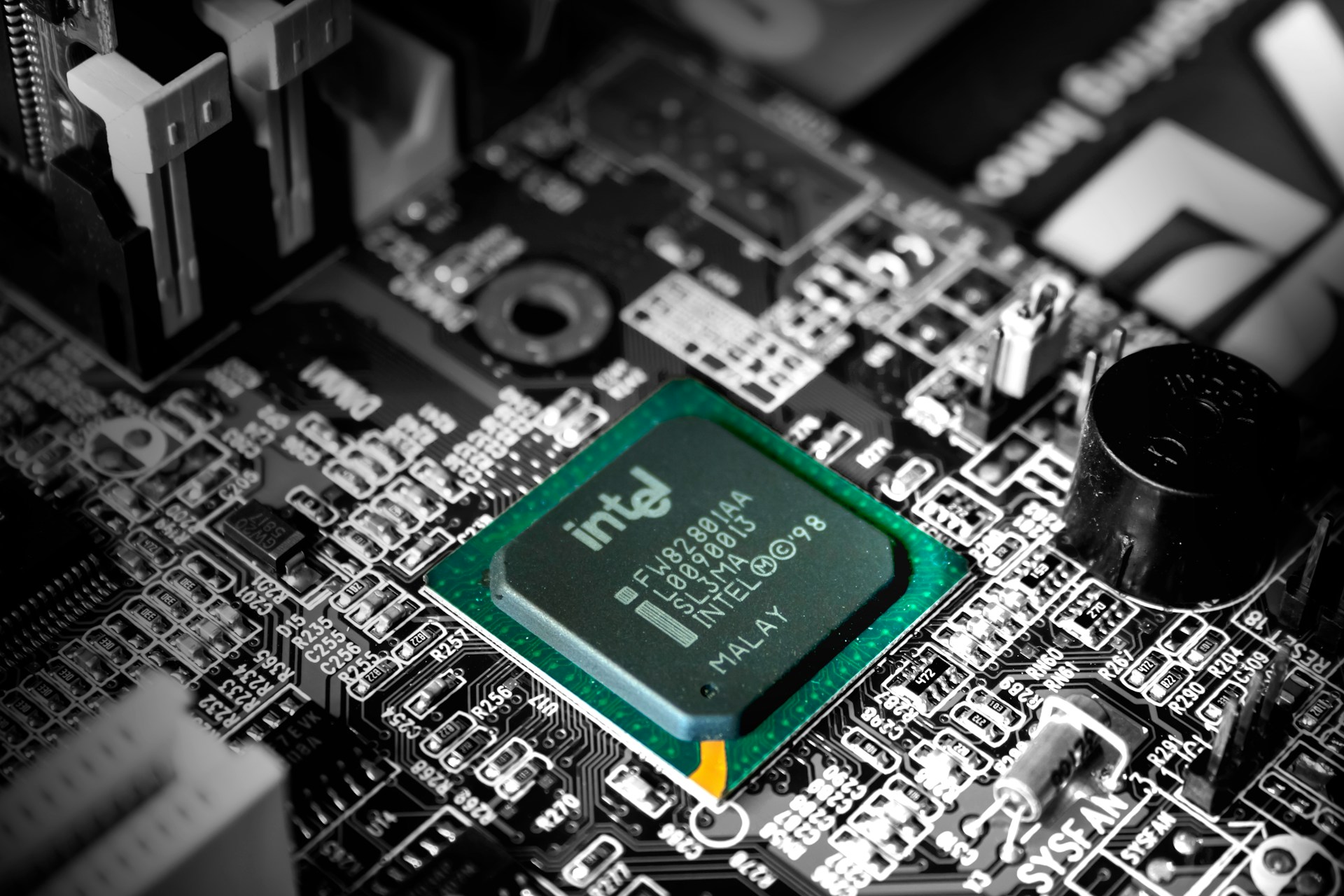
The Intel 8086, released in 1978, marked a significant milestone in the x86 processor family. It introduced a 16-bit architecture, paving the way for powerful software development. Let’s explore its intricacies!
8086: The First x86 Processor
The Intel 8086 was the first 16-bit microprocessor in the x86 family. Here are some key points about it:
- 16-Bit Architecture: The 8086 operated on 16-bit data and addresses, allowing for larger memory access.
- Instruction Set: It had a rich instruction set, including arithmetic, logical, and control instructions.
- Clock Speed: The original 8086 ran at 5 MHz, but later versions reached higher speeds.
8086 Processor Architecture
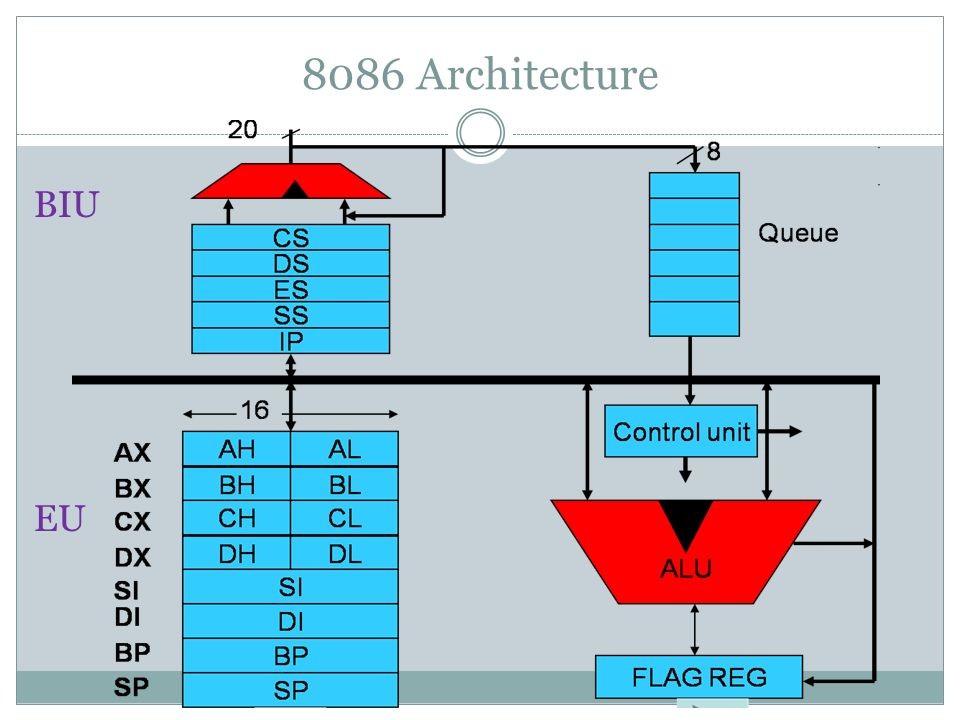
ALU (Arithmetic Logic Unit)
- Responsible for performing arithmetic and logical operations.
- Supports addition, subtraction, multiplication, division, and bitwise operations.
Control Unit
- Manages instruction execution.
- Decodes instructions and coordinates data movement.
Bus Interface Unit (BIU) and Execution Unit (EU)
- BIU fetches instructions from memory.
- EU executes instructions, including ALU operations.
Memory Segmentation
- The 8086 uses segmentation to address memory.
- Each segment is 64KB, and the combination of segment and offset forms a 20-bit physical address.
8086 Registers
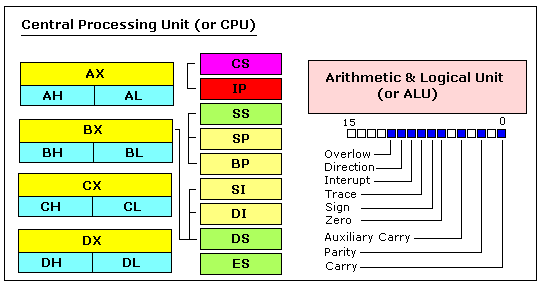
General Registers
The 8086 has four general-purpose registers:
- AX (Accumulator): Used for arithmetic operations.
- BX (Base Register): Often used for addressing data.
- CX (Count Register): Used for loops and string operations.
- DX (Data Register): Used for I/O operations.
Segment Registers
Segment registers include:
- CS (Code Segment): Points to the code segment.
- DS (Data Segment): Points to the data segment.
- SS (Stack Segment): Manages the stack.
- ES (Extra Segment): Additional data segment.
Special Purpose Registers
- IP (Instruction Pointer): Holds the address of the next instruction.
- SP (Stack Pointer): Points to the top of the stack.
- BP (Base Pointer): Used for stack frame management.
- SI (Source Index) and DI (Destination Index): Assist in string operations.
Flag Register
This is a single designated register that stores all the flags that one might use during operations. We’ll cover them right below.
Flags (Flag Registers)
The Intel 8086 processor has several flags that provide information about the outcome of operations. These flags are essential for decision-making and conditional branching in assembly language programming. Here’s a comprehensive list:
- Zero Flag (ZF):
- Description: Set if the result of an operation is zero.
- Usage Example:
MOV AX, 0
CMP AX, 0
JZ ZeroDetected ; Jump if ZF is set
- Sign Flag (SF):
- Description: Set if the result is negative (i.e., the most significant bit is 1).
- Usage Example:
MOV AX, -1
TEST AX, AX
JS NegativeDetected ; Jump if SF is set
- Overflow Flag (OF):
- Description: Indicates overflow in signed arithmetic.
- Usage Example (signed addition):
MOV AX, 32767
ADD AX, 1
JO OverflowDetected ; Jump if OF is set
- Carry Flag (CF):
- Description: Indicates carry or borrow.
- Usage Example (unsigned subtraction):
MOV AX, 10
SUB AX, 20
JC CarryDetected ; Jump if CF is set
-
Auxiliary Carry Flag (AF):
- Description: Used in binary-coded decimal (BCD) arithmetic.
- Not commonly used in high-level programming.
-
Parity Flag (PF):
- Description: Set if the result has an even number of set (1) bits.
- Rarely used in modern programming due to byte-oriented nature.
-
Reserved Flags (RF, IF, TF, DF, OF, NT):
- Reserved for system use.
- Not directly manipulated by programmers.
Floating Point
The original 8086 lacked native floating-point support. However, there were ways to perform floating-point operations:
- 8087 Coprocessor (FPU):
- The 8087 was an optional coprocessor specifically designed to handle floating-point arithmetic.
- It provided instructions like
FADD
,FSUB
, andFMUL
. - If absent, the 8086 would trap these instructions, allowing software emulation.
- Software Emulation:
- Routines used integer arithmetic to simulate floating-point behavior.
- Fixed-point representations were common.
- Modern Context:
- CPUs now use SSE and SSE2 for floating-point math.
- Emulators like DOSBox or BOCHS can simulate the 8087 FPU for legacy software.
The 8086’s legacy lives on in modern CPUs, compilers, and operating systems!
Segmentation and Addressing
Segmentation:
- Memory Segmentation: The 8086 uses a memory segmentation model to address memory. Instead of a flat memory space, it divides memory into four segments.
- Segment Size: Each segment is 64KB (kilobytes) in size.
- Purpose: Segmentation allows the 8086 to access more memory than a straightforward 16-bit address space would allow.
- Segment Registers: The processor has segment registers (CS, DS, SS, ES) that point to different segments.
- CS (Code Segment): Points to the segment containing executable code.
- DS (Data Segment): Points to the segment containing data.
- SS (Stack Segment): Manages the stack.
- ES (Extra Segment): An additional data segment.
Addressing:
- Logical Address: In the 8086, an address consists of two parts:
- Segment: A 16-bit value from a segment register.
- Offset: A 16-bit value representing the position within the segment.
- Physical Address: The combination of the segment and offset forms a 20-bit physical address.
- Calculation: The actual physical address is obtained by shifting the segment value left by 4 bits (effectively multiplying it by 16) and adding the offset value.
Example:
Suppose we have the following:
- CS = 0x1000 (Code Segment)
- IP (Instruction Pointer) = 0x0010 (Offset)
The logical address is 0x1000:0x0010. To calculate the physical address:
- Shift the segment value (0x1000) left by 4 bits: 0x10000.
- Add the offset value (0x0010): 0x10010.
The physical address is 0x10010.
Segmentation was a unique feature of the 8086, and understanding it is essential for effective memory management and programming! 🧠 🔍
Endianness
The 8086 is little-endian, meaning it stores the least significant byte first in memory.
8086 Backward and Forward Compatibility
The 8086’s architecture influenced subsequent x86 processors. It remains backward compatible, allowing older software to run on modern systems.
Conclusion
The Intel 8086 revolutionized computing, shaping the x86 architecture we know today. Its legacy lives on in modern CPUs, compilers, and operating systems.
FAQ
Is 8086 Big Endian or Small Endian?
The 8086 is little-endian.
Is 8086 Still Used Today?
While not in active use, emulators and historical enthusiasts keep the 8086 alive.
Is There an Emulator for 8086?
Yes, EMU8086 is a popular emulator for running 8086 assembly code on modern systems.